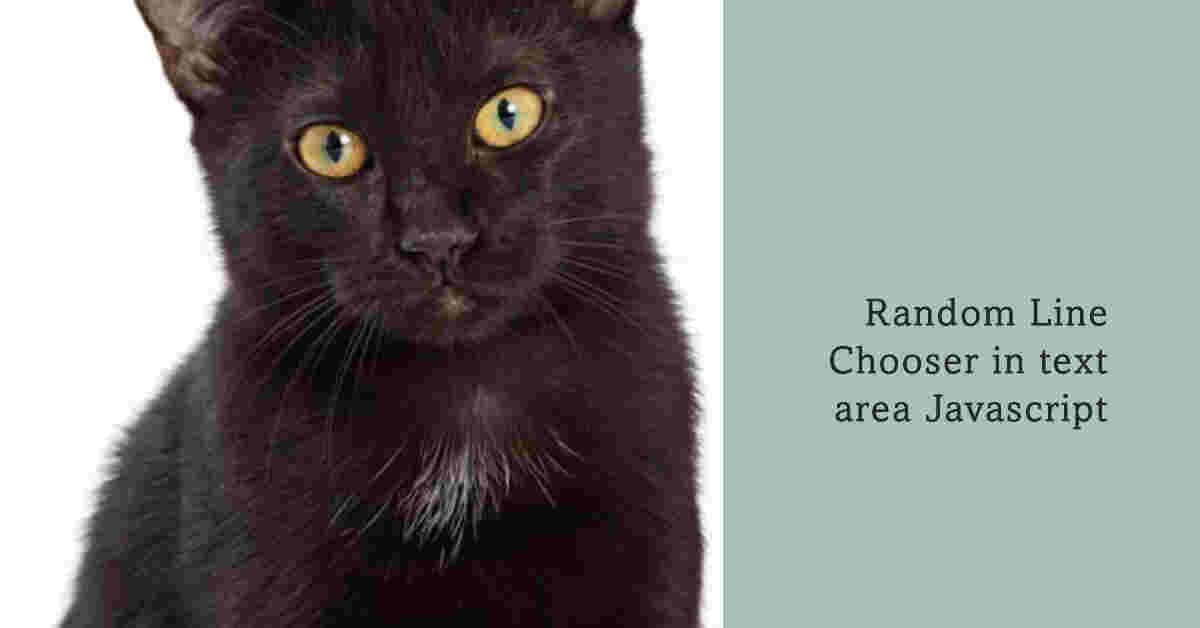
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Random Line Chooser</title>
<script type="text/javascript">
function copyToClipboard() {
const textarea = document.getElementById("text-area");
const lines = textarea.value.split("\n").filter(line => line.trim() !== "");
const randomIndex = Math.floor(Math.random() * lines.length);
navigator.clipboard.writeText(lines[randomIndex]);
// Save last edited text area to localStorage
localStorage.setItem("last-edited-text", textarea.value);
}
// Load last edited text area from localStorage on page load
window.onload = function() {
const textarea = document.getElementById("text-area");
const lastEditedText = localStorage.getItem("last-edited-text");
if (lastEditedText) {
textarea.value = lastEditedText;
}
};
</script>
</head>
<body>
<h1>Random Line Chooser</h1>
<textarea id="text-area">Enter some text here, with each line being a possible choice.</textarea>
<br>
<button onclick="copyToClipboard()">Choose a random line and copy it to clipboard</button>
</body>
</html>
result: